API Documentation
Error Codes
{
"status":false,
"errno":94,
"errmsg":"invalid or missing key",
}
errno | errmsg | Description |
---|---|---|
50 | access denied | Unauthorized IP Address or Referer. Please check your access privileges. |
90 | invalid country code | Check supported country codes. ISO 3166-1 alpha-2 |
91 | name not set || email not set | Missing name or email parameter on your request. |
92 | too many names || too many emails | Limit is 100 for names, 50 for emails in one request. |
93 | limit reached | The API key credit has been finished. |
94 | invalid or missing key | The API key cannot be found. |
99 | API key has expired | Please renew your API key. |
Postman
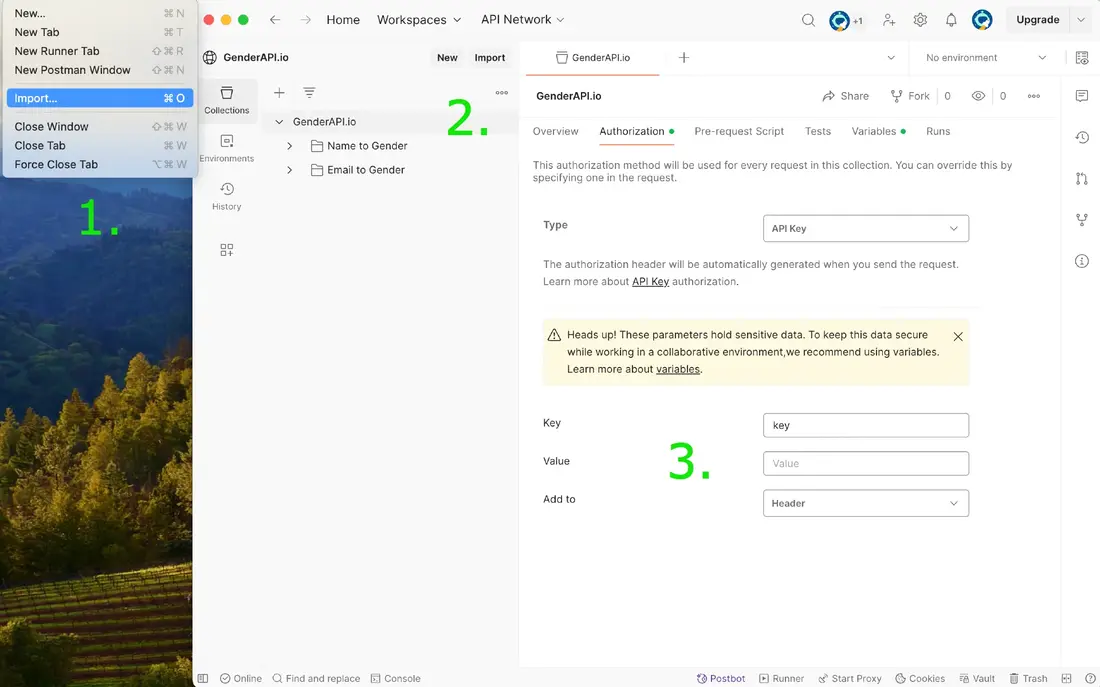
Name to Gender
Single Name
Basic usage of single name request
GET /api/?name=Anna&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
name=Anna&key=<YourAPIkey>
Select Your Programing Language
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => 'name=abraham&key=<YourAPIkey>',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'name' => 'abraham',
'key' => '<YourAPIkey>'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'name' => 'maria',
'key' => '<YourAPIkey>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' . $response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'name' => 'maria',
'key' => '<YourAPIkey>')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/?name=abraham&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = 'name=maria&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/"
payload = 'name=maria&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "name=maria&key=<YourAPIkey>");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>")
.asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("name", "maria")
.field("key", "<YourAPIkey>")
.asString();
var requestOptions = {
method: 'GET',
redirect: 'follow'
};
fetch("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
POST
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
var urlencoded = new URLSearchParams();
urlencoded.append("name", "maria");
urlencoded.append("key", "<YourAPIkey>");
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: urlencoded,
redirect: 'follow'
};
fetch("https://api.genderapi.io/api/", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
var settings = {
"url": "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"name": "maria",
"key": "<YourAPIkey>"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "name=maria&key=<YourAPIkey>";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/");
var collection = new List<KeyValuePair<string, string>>();
collection.Add(new("name", "maria"));
collection.Add(new("key", "<YourAPIkey>"));
var content = new FormUrlEncodedContent(collection);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/?name=abraham&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("name", "maria");
request.AddParameter("key", "<YourAPIkey>");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/?name=abraham&key=<Your-API-Key>'
POST
curl --location 'https://api.genderapi.io/api/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'name=maria' \
--data-urlencode 'key=<YourAPIkey>'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'name': 'maria',
'key': '<YourAPIkey>'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/'));
request.bodyFields = {
'name': 'maria',
'key': '<YourAPIkey>'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>"
method := "GET"
client := &http.Client {}
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/"
method := "POST"
payload := strings.NewReader("name=maria&key=<YourAPIkey>")
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "name=maria&key=<YourAPIkey>".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "name=maria&key=<YourAPIkey>";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'name': 'maria',
'key': '<YourAPIkey>'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/?name=abraham&key=<YourAPIkey>',
'headers': {},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'name': 'maria',
'key': '<YourAPIkey>'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>',
'headers': {}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'name': 'maria',
'key': '<YourAPIkey>'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('name=maria')
.send('key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"name=maria" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "name=maria&key=<YourAPIkey>";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$response = Invoke-RestMethod 'https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "name=maria&key=<YourAPIkey>"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'name' = 'maria',
'key' = '<YourAPIkey>'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"name" = "maria",
"key" = "<YourAPIkey>"
)
res <- postForm("https://api.genderapi.io/api/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "name=maria&key=<YourAPIkey>"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/?name=abraham&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("name", "maria");
params.insert("key", "<YourAPIkey>");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/?name=Anna&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "name=abraham&key=<YourAPIkey>"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/?name=Anna&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/' \
'name'='abraham' \
'key'='<YourAPIkey>' \
Content-Type:'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/?name=Anna&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data 'name=abraham&key=<YourAPIkey>' \
'https://api.genderapi.io/api/'
RESPONSE
{
"status": true,
"used_credits": 1,
"remaining_credits": 7295,
"expires": 1717069765,
"q": "anna",
"name": "anna",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99,
"duration": "4ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
duration | String | milliseconds "4ms" | Processing time of your request on server |
Single Name with Country Filter
In one country, a name can be female while in another country, it can be male.
By adding the country filter to your queries, you can obtain gender information specific to that country.
Find the ISO 3166-1 alpha-2 country codes
* If there is no result with given country filter, we will give the global name result.
GET /api/?name=Anna&country=US&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
name=Anna&country=US&key=<YourAPIkey>
Select Your Programing Language
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => 'name=Anna&key=<YourAPIkey>&country=US',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'name' => 'Anna',
'key' => '<YourAPIkey>',
'country' => 'US'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'name' => 'Anna',
'key' => '<YourAPIkey>',
'country' => 'US'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'name' => 'Anna',
'key' => '<YourAPIkey>',
'country' => 'US')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/?name=Anna&country=US&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = 'name=Anna&key=<YourAPIkey>&country=US'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/"
payload = 'name=Anna&key=<YourAPIkey>&country=US'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "name=Anna&key=<YourAPIkey>&country=US");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>").asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("name", "Anna")
.field("key", "<YourAPIkey>")
.field("country", "US")
.asString();
const requestOptions = {
method: "GET",
redirect: "follow"
};
fetch("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
POST
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
const urlencoded = new URLSearchParams();
urlencoded.append("name", "Anna");
urlencoded.append("key", "<YourAPIkey>");
urlencoded.append("country", "US");
const requestOptions = {
method: "POST",
headers: myHeaders,
body: urlencoded,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"name": "Anna",
"key": "<YourAPIkey>",
"country": "US"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "name=Anna&key=<YourAPIkey>&country=US";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/");
var collection = new List<KeyValuePair<string, string>>();
collection.Add(new("name", "Anna"));
collection.Add(new("key", "<YourAPIkey>"));
collection.Add(new("country", "US"));
var content = new FormUrlEncodedContent(collection);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("name", "Anna");
request.AddParameter("key", "<YourAPIkey>");
request.AddParameter("country", "US");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>'
POST
curl --location 'https://api.genderapi.io/api/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'name=Anna' \
--data-urlencode 'key=<YourAPIkey>' \
--data-urlencode 'country=US'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'name': 'Anna',
'key': '<YourAPIkey>',
'country': 'US'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/'));
request.bodyFields = {
'name': 'Anna',
'key': '<YourAPIkey>',
'country': 'US'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>"
method := "GET"
client := &http.Client { }
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/"
method := "POST"
payload := strings.NewReader("name=Anna&key=<YourAPIkey>&country=US")
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "name=Anna&key=<YourAPIkey>&country=US".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "name=Anna&key=<YourAPIkey>&country=US";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'name': 'Anna',
'key': '<YourAPIkey>',
'country': 'US'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/?name=Anna&country=US&key=<YourAPIkey>',
'headers': {},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'name': 'Anna',
'key': '<YourAPIkey>',
'country': 'US'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>',
'headers': { }
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'name': 'Anna',
'key': '<YourAPIkey>',
'country': 'US'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('name=Anna')
.send('key=<YourAPIkey>')
.send('country=US')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"name=Anna" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&country=US" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "name=Anna&key=<YourAPIkey>&country=US";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$response = Invoke-RestMethod 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "name=Anna&key=<YourAPIkey>&country=US"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'name' = 'Anna',
'key' = '<YourAPIkey>',
'country' = 'US'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"name" = "Anna",
"key" = "<YourAPIkey>",
"country" = "US"
)
res <- postForm("https://api.genderapi.io/api/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "name=Anna&key=<YourAPIkey>&country=US"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("name", "Anna");
params.insert("key", "<YourAPIkey>");
params.insert("country", "US");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/' \
'name'='Anna' \
'key'='<YourAPIkey>' \
'country'='US' \
'Content-Type':'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data 'name=Anna&key=<YourAPIkey>&country=US' \
'https://api.genderapi.io/api/'
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/?name=Anna&country=US&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "name=Anna&key=<YourAPIkey>&country=US"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 1,
"remaining_credits": 7295,
"expires": 1717069765,
"q": "anna",
"name": "anna",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99,
"duration": "4ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
duration | String | milliseconds "4ms" | Processing time of your request on server |
Multiple Names
You can query multiple names at once. The maximum limit is 100.
GET /api/?name[]=Anna&name[]=james&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
name=Anna&name=james&key=<YourAPIkey>
Select Your Programing Language
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("name", "Anna");
request.AddParameter("name", "james");
request.AddParameter("key", "<YourAPIkey>");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location --globoff 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>'
POST
curl --location 'https://api.genderapi.io/api/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'name=Anna' \
--data-urlencode 'name=james' \
--data-urlencode 'key=<YourAPIkey>'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'name': 'Anna',
'name': 'james',
'key': '<YourAPIkey>'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/'));
request.bodyFields = {
'name': 'Anna',
'name': 'james',
'key': '<YourAPIkey>'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>"
method := "GET"
client := &http.Client { }
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/"
method := "POST"
payload := strings.NewReader("name=Anna&name=james&key=<YourAPIkey>")
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "name=Anna&name=james&key=<YourAPIkey>");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")
.asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("name", "Anna")
.field("name", "james")
.field("key", "<YourAPIkey>")
.asString();
const requestOptions = {
method: "GET",
redirect: "follow"
};
fetch("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
POST
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
const urlencoded = new URLSearchParams();
urlencoded.append("name", "Anna");
urlencoded.append("name", "james");
urlencoded.append("key", "<YourAPIkey>");
const requestOptions = {
method: "POST",
headers: myHeaders,
body: urlencoded,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"name": "Anna",
"name": "james",
"key": "<YourAPIkey>"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "name=Anna&name=james&key=<YourAPIkey>";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "name=Anna&name=james&key=<YourAPIkey>".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "name=Anna&name=james&key=<YourAPIkey>";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'name': 'Anna',
'name': 'james',
'key': '<YourAPIkey>'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/?name%5B%5D=Anna&name%5B%5D=james&key=<YourAPIkey>',
'headers': { },
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'name': 'Anna',
'name': 'james',
'key': '<YourAPIkey>'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>',
'headers': { }
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'name': 'Anna',
'name': 'james',
'key': '<YourAPIkey>'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('name=Anna')
.send('name=james')
.send('key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/?name%5B%5D=Anna&name%5B%5D=james&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"name=Anna" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&name=james" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "name=Anna&name=james&key=<YourAPIkey>";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => 'name=Anna&name=james&key=<YourAPIkey>',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
code>
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'name' => 'Anna',
'name' => 'james',
'key' => '<YourAPIkey>'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'name' => 'Anna',
'name' => 'james',
'key' => '<YourAPIkey>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'name' => 'Anna',
'name' => 'james',
'key' => '<YourAPIkey>')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
$response = Invoke-RestMethod 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "name=Anna&name=james&key=<YourAPIkey>"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/?name%5B%5D=Anna&name%5B%5D=james&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = 'name=Anna&name=james&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/"
payload = 'name=Anna&name=james&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'name' = 'Anna',
'name' = 'james',
'key' = '<YourAPIkey>'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"name" = "Anna",
"name" = "james",
"key" = "<YourAPIkey>"
)
res <- postForm("https://api.genderapi.io/api/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "name=Anna&name=james&key=<YourAPIkey>"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("name", "Anna");
params.insert("name", "james");
params.insert("key", "<YourAPIkey>");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/' \
'name'='Anna' \
'name'='james' \
'key'='<YourAPIkey>' \
Content-Type:'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data 'name=Anna&name=james&key=<YourAPIkey>' \
'https://api.genderapi.io/api/'
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/?name[]=Anna&name[]=james&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "name=Anna&name=james&key=<YourAPIkey>"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 2,
"remaining_credits": 7291,
"expires": 1717069765,
"q": "anna;james brown",
"names": [
{
"name": "james",
"q": "james brown",
"gender": "male",
"country": "US",
"total_names": 70373,
"probability": 99
},
{
"name": "anna",
"q": "anna",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99
}
],
"duration": "2ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
names | Array | Any | List of your results |
duration | String | milliseconds("4ms") | Processing time of your request on server |
names(Array) | |||
---|---|---|---|
Field | Data Type | Value | Description |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
Multiple Names with Country Filter
In one country, a name can be female while in another country, it can be male.
By adding the country filter to your queries, you can obtain gender information specific to that country.
Find the ISO 3166-1 alpha-2 country codes
* If there is no result with given country filter, we will give the global name result.
* You can query multiple names at once. The maximum limit is 100.
POST /api/name/multi/country HTTP/1.1
Host: api.genderapi.io
Content-Type: application/json
{
"key":"<YourAPIkey>",
"data":[
{"name":"Andrea","country":"DE"},
{"name":"andrea","country":"IT"},
{"name":"james","country":"US"}
]
}
Select Your Programing Language
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/name/multi/country");
var content = new StringContent("{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}", null, "application/json");
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/name/multi/country", Method.Post);
request.AddHeader("Content-Type", "application/json");
var body = @"{" + "\n" +
@" ""key"":""YourApiKey""," + "\n" +
@" ""data"":[{""name"":""Andrea"",""country"":""DE""},{""name"":""andrea"",""country"":""IT""},{""name"":""james"",""country"":""US""}]" + "\n" +
@"}";
request.AddStringBody(body, DataFormat.Json);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/name/multi/country' \
--header 'Content-Type: application/json' \
--data '{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}'
var headers = {
'Content-Type': 'application/json'
};
var data = json.encode({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/name/multi/country',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var headers = {
'Content-Type': 'application/json'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/name/multi/country'));
request.body = json.encode({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/name/multi/country"
method := "POST"
payload := strings.NewReader(`{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}`)
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/json")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/name/multi/country")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/name/multi/country")
.header("Content-Type", "application/json")
.body("{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}")
.asString();
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
const raw = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
const requestOptions = {
method: "POST",
headers: myHeaders,
body: raw,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/name/multi/country", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/name/multi/country",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/json"
},
"data": JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For POST requests, body is set to null by browsers.
var data = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/name/multi/country");
xhr.setRequestHeader("Content-Type", "application/json");
xhr.send(data);
val client = OkHttpClient()
val mediaType = "application/json".toMediaType()
val body = "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/name/multi/country")
.post(body)
.addHeader("Content-Type", "application/json")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/name/multi/country");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/json");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "{\n \"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let data = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/name/multi/country',
headers: {
'Content-Type': 'application/json'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/name/multi/country',
'headers': {
'Content-Type': 'application/json'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/name/multi/country',
'headers': {
'Content-Type': 'application/json'
},
body: JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/name/multi/country')
.headers({
'Content-Type': 'application/json'
})
.send(JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
}))
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/name/multi/country"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/json"
};
[request setAllHTTPHeaderFields:headers];
NSData *postData = [[NSData alloc] initWithData:[@"{\n \"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/name/multi/country" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/json"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/name/multi/country',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$headers = [
'Content-Type' => 'application/json'
];
$body = '{
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
}';
$request = new Request('POST', 'https://api.genderapi.io/api/name/multi/country', $headers, $body);
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/name/multi/country');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json'
));
$request->setBody('{\n "key":"<YourAPIkey>",\n "data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/name/multi/country');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/json")
$body = @"
{
`"key`":`"<YourAPIkey>`",
`"data`":[{`"name`":`"Andrea`",`"country`":`"DE`"},{`"name`":`"andrea`",`"country`":`"IT`"},{`"name`":`"james`",`"country`":`"US`"}]
}
"@
$response = Invoke-RestMethod 'https://api.genderapi.io/api/name/multi/country' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
import http.client
import json
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = json.dumps({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
})
headers = {
'Content-Type': 'application/json'
}
conn.request("POST", "/api/name/multi/country", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
import json
url = "https://api.genderapi.io/api/name/multi/country"
payload = json.dumps({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
library(httr)
headers = c(
'Content-Type' = 'application/json'
)
body = '{
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
}';
res <- VERB("POST", url = "https://api.genderapi.io/api/name/multi/country", body = body, add_headers(headers))
cat(content(res, 'text'))
library(RCurl)
headers = c(
"Content-Type" = "application/json"
)
params = "{
\"key\": \"<YourAPIkey>\",
\"data\": [
{
\"name\": \"Andrea\",
\"country\": \"DE\"
},
{
\"name\": \"andrea\",
\"country\": \"IT\"
},
{
\"name\": \"james\",
\"country\": \"US\"
}
]
}"
res <- postForm("https://api.genderapi.io/api/name/multi/country", .opts=list(postfields = params, httpheader = headers, followlocation = TRUE), style = "httppost")
cat(res)
require "uri"
require "json"
require "net/http"
url = URI("https://api.genderapi.io/api/name/multi/country")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/json"
request.body = JSON.dump({
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
})
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/json".parse()?);
let data = r#"{
"key": "<YourAPIkey>",
"data": [
{
"name": "Andrea",
"country": "DE"
},
{
"name": "andrea",
"country": "IT"
},
{
"name": "james",
"country": "US"
}
]
}"#;
let json: serde_json::Value = serde_json::from_str(&data)?;
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/name/multi/country")
.headers(headers)
.json(&json);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
printf '{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}'| http --follow --timeout 3600 POST 'https://api.genderapi.io/api/name/multi/country' \
Content-Type:'application/json'
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/json' \
--body-data '{
"key":"<YourAPIkey>",
"data":[{"name":"Andrea","country":"DE"},{"name":"andrea","country":"IT"},{"name":"james","country":"US"}]
}' \
'https://api.genderapi.io/api/name/multi/country'
let parameters = "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"name\":\"Andrea\",\"country\":\"DE\"},{\"name\":\"andrea\",\"country\":\"IT\"},{\"name\":\"james\",\"country\":\"US\"}]\n}"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/name/multi/country")!,timeoutInterval: Double.infinity)
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 3,
"remaining_credits": 7265,
"expires": 1717069765,
"names": [
{
"name": "andrea",
"q": "Andrea",
"gender": "female",
"country": "DE",
"total_names": 644,
"probability": 88
},
{
"name": "andrea",
"q": "andrea",
"gender": "male",
"country": "IT",
"total_names": 13537,
"probability": 98
},
{
"name": "james",
"q": "james",
"gender": "male",
"country": "US",
"total_names": 45274,
"probability": 100
}
],
"duration": "5ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
names | Array | Any | List of your results |
duration | String | milliseconds("4ms") | Processing time of your request on server |
names(Array) | |||
---|---|---|---|
Field | Data Type | Value | Description |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
Email to Gender
Single Email
Basic usage of single email request
GET /api/email/[email protected]&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/email/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
[email protected]&key=<YourAPIkey>
Select Your Programing Language
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => '[email protected]&key=<YourAPIkey>',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'email' => '[email protected]',
'key' => '<YourAPIkey>'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/email/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'email' => '[email protected]',
'key' => '<YourAPIkey>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'email' => '[email protected]',
'key' => '<YourAPIkey>')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/email/[email protected]&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = '[email protected]&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/email/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/email/"
payload = '[email protected]&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "[email protected]&key=<YourAPIkey>");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")
.asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/email/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("email", "[email protected]")
.field("key", "<YourAPIkey>")
.asString();
var requestOptions = {
method: 'GET',
redirect: 'follow'
};
fetch("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
POST
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
var urlencoded = new URLSearchParams();
urlencoded.append("email", "[email protected]");
urlencoded.append("key", "<YourAPIkey>");
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: urlencoded,
redirect: 'follow'
};
fetch("https://api.genderapi.io/api/email/", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
var settings = {
"url": "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/email/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"email": "[email protected]",
"key": "<YourAPIkey>"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "[email protected]&key=<YourAPIkey>";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/email/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/email/");
var collection = new List<KeyValuePair<string, string>>();
collection.Add(new("email", "[email protected]"));
collection.Add(new("key", "<YourAPIkey>"));
var content = new FormUrlEncodedContent(collection);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/email/[email protected]&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/email/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("email", "[email protected]");
request.AddParameter("key", "<YourAPIkey>");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/email/[email protected]&key=<Your-API-Key>'
POST
curl --location 'https://api.genderapi.io/api/email/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode '[email protected]' \
--data-urlencode 'key=<YourAPIkey>'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'email': '[email protected]',
'key': '<YourAPIkey>'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/email/'));
request.bodyFields = {
'email': '[email protected]',
'key': '<YourAPIkey>'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>"
method := "GET"
client := &http.Client {}
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/"
method := "POST"
payload := strings.NewReader("[email protected]&key=<YourAPIkey>")
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "[email protected]&key=<YourAPIkey>".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "[email protected]&key=<YourAPIkey>";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'email': '[email protected]',
'key': '<YourAPIkey>'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/email/[email protected]&key=<YourAPIkey>',
'headers': {},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'email': '[email protected]',
'key': '<YourAPIkey>'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>',
'headers': {}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'email': '[email protected]',
'key': '<YourAPIkey>'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/email/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('[email protected]')
.send('key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"[email protected]" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "[email protected]&key=<YourAPIkey>";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "[email protected]&key=<YourAPIkey>"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'email' = '[email protected]',
'key' = '<YourAPIkey>'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/email/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"email" = "[email protected]",
"key" = "<YourAPIkey>"
)
res <- postForm("https://api.genderapi.io/api/email/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "[email protected]&key=<YourAPIkey>"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("email", "[email protected]");
params.insert("key", "<YourAPIkey>");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/email/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "[email protected]&key=<YourAPIkey>"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/email/' \
'email'='[email protected]' \
'key'='<YourAPIkey>' \
Content-Type:'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/email/[email protected]&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data '[email protected]&key=<YourAPIkey>' \
'https://api.genderapi.io/api/email/'
RESPONSE
{
"status": true,
"used_credits": 1,
"remaining_credits": 4,
"expires": 1717046468,
"q": "[email protected]",
"name": "anna",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99,
"duration": "11ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
duration | String | milliseconds "4ms" | Processing time of your request on server |
Single Email with Country Filter
In one country, a name can be female while in another country, it can be male.
By adding the country filter to your queries, you can obtain gender information specific to that country.
Find the ISO 3166-1 alpha-2 country codes
* If there is no result with given country filter, we will give the global name result.
GET /api/email/[email protected]&country=US&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/email/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
[email protected]&country=US&key=<YourAPIkey>
Select Your Programing Language
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => '[email protected]&key=<YourAPIkey>&country=US',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'email' => '[email protected]',
'key' => '<YourAPIkey>',
'country' => 'US'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/email/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'email' => '[email protected]',
'key' => '<YourAPIkey>',
'country' => 'US'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'email' => '[email protected]',
'key' => '<YourAPIkey>',
'country' => 'US')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/email/[email protected]&country=US&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = '[email protected]&key=<YourAPIkey>&country=US'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/email/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/email/"
payload = '[email protected]&key=<YourAPIkey>&country=US'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "[email protected]&key=<YourAPIkey>&country=US");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>").asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/email/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("email", "[email protected]")
.field("key", "<YourAPIkey>")
.field("country", "US")
.asString();
const requestOptions = {
method: "GET",
redirect: "follow"
};
fetch("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
POST
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
const urlencoded = new URLSearchParams();
urlencoded.append("email", "[email protected]");
urlencoded.append("key", "<YourAPIkey>");
urlencoded.append("country", "US");
const requestOptions = {
method: "POST",
headers: myHeaders,
body: urlencoded,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/email/", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/email/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"email": "[email protected]",
"key": "<YourAPIkey>",
"country": "US"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "[email protected]&key=<YourAPIkey>&country=US";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/email/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/email/");
var collection = new List<KeyValuePair<string, string>>();
collection.Add(new("email", "[email protected]"));
collection.Add(new("key", "<YourAPIkey>"));
collection.Add(new("country", "US"));
var content = new FormUrlEncodedContent(collection);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/email/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("email", "[email protected]");
request.AddParameter("key", "<YourAPIkey>");
request.AddParameter("country", "US");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>'
POST
curl --location 'https://api.genderapi.io/api/email/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode '[email protected]' \
--data-urlencode 'key=<YourAPIkey>' \
--data-urlencode 'country=US'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'email': '[email protected]',
'key': '<YourAPIkey>',
'country': 'US'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/email/'));
request.bodyFields = {
'email': '[email protected]',
'key': '<YourAPIkey>',
'country': 'US'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>"
method := "GET"
client := &http.Client { }
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/"
method := "POST"
payload := strings.NewReader("[email protected]&key=<YourAPIkey>&country=US")
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "[email protected]&key=<YourAPIkey>&country=US".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "[email protected]&key=<YourAPIkey>&country=US";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'email': '[email protected]',
'key': '<YourAPIkey>',
'country': 'US'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/email/[email protected]&country=US&key=<YourAPIkey>',
'headers': {},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'email': '[email protected]',
'key': '<YourAPIkey>',
'country': 'US'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>',
'headers': { }
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'email': '[email protected]',
'key': '<YourAPIkey>',
'country': 'US'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/email/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('[email protected]')
.send('key=<YourAPIkey>')
.send('country=US')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"[email protected]" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&country=US" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "[email protected]&key=<YourAPIkey>&country=US";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "[email protected]&key=<YourAPIkey>&country=US"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'email' = '[email protected]',
'key' = '<YourAPIkey>',
'country' = 'US'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/email/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"email" = "[email protected]",
"key" = "<YourAPIkey>",
"country" = "US"
)
res <- postForm("https://api.genderapi.io/api/email/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "[email protected]&key=<YourAPIkey>&country=US"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("email", "[email protected]");
params.insert("key", "<YourAPIkey>");
params.insert("country", "US");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/email/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/email/' \
'email'='[email protected]' \
'key'='<YourAPIkey>' \
'country'='US' \
'Content-Type':'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data '[email protected]&key=<YourAPIkey>&country=US' \
'https://api.genderapi.io/api/email/'
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/[email protected]&country=US&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "[email protected]&key=<YourAPIkey>&country=US"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 1,
"remaining_credits": 4,
"expires": 1717046468,
"q": "[email protected]",
"name": "anna",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99,
"duration": "11ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
duration | String | milliseconds "4ms" | Processing time of your request on server |
Multiple Emails
You can query multiple emails at once. The maximum limit is 50.
GET /api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey> HTTP/1.1
Host: api.genderapi.io
POST /api/email/ HTTP/1.1
Host: api.genderapi.io
Content-Type: application/x-www-form-urlencoded
[email protected][email protected]&key=<YourAPIkey>
Select Your Programing Language
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Get, "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>");
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
POST
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>", Method.Get);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
POST
var options = new RestClientOptions("")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("https://api.genderapi.io/api/email/", Method.Post);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("email", "[email protected]");
request.AddParameter("email", "[email protected]");
request.AddParameter("key", "<YourAPIkey>");
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location --globoff 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>'
POST
curl --location 'https://api.genderapi.io/api/email/' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode '[email protected]' \
--data-urlencode '[email protected]' \
--data-urlencode 'key=<YourAPIkey>'
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>',
options: Options(
method: 'GET',
),
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var data = {
'email': '[email protected]',
'email': '[email protected]',
'key': '<YourAPIkey>'
};
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var request = http.Request('GET', Uri.parse('https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>'));
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
POST
var headers = {
'Content-Type': 'application/x-www-form-urlencoded'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/email/'));
request.bodyFields = {
'email': '[email protected]',
'email': '[email protected]',
'key': '<YourAPIkey>'
};
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>"
method := "GET"
client := &http.Client { }
req, err := http.NewRequest(method, url, nil)
if err != nil {
fmt.Println(err)
return
}
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
POST
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/"
method := "POST"
payload := strings.NewReader("[email protected][email protected]&key=<YourAPIkey>")
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("text/plain");
RequestBody body = RequestBody.create(mediaType, "");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")
.method("GET", body)
.build();
Response response = client.newCall(request).execute();
POST
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/x-www-form-urlencoded");
RequestBody body = RequestBody.create(mediaType, "[email protected][email protected]&key=<YourAPIkey>");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/")
.method("POST", body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.get("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")
.asString();
POST
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/email/")
.header("Content-Type", "application/x-www-form-urlencoded")
.field("email", "[email protected]")
.field("email", "[email protected]")
.field("key", "<YourAPIkey>")
.asString();
const requestOptions = {
method: "GET",
redirect: "follow"
};
fetch("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
POST
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/x-www-form-urlencoded");
const urlencoded = new URLSearchParams();
urlencoded.append("email", "[email protected]");
urlencoded.append("email", "[email protected]");
urlencoded.append("key", "<YourAPIkey>");
const requestOptions = {
method: "POST",
headers: myHeaders,
body: urlencoded,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/email/", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>",
"method": "GET",
"timeout": 0,
};
$.ajax(settings).done(function (response) {
console.log(response);
});
POST
var settings = {
"url": "https://api.genderapi.io/api/email/",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"email": "[email protected]",
"email": "[email protected]",
"key": "<YourAPIkey>"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For GET requests, body is set to null by browsers.
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>");
xhr.send();
POST
// WARNING: For POST requests, body is set to null by browsers.
var data = "[email protected][email protected]&key=<YourAPIkey>";
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/email/");
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(data);
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")
.build()
val response = client.newCall(request).execute()
POST
val client = OkHttpClient()
val mediaType = "application/x-www-form-urlencoded".toMediaType()
val body = "[email protected][email protected]&key=<YourAPIkey>".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/")
.post(body)
.addHeader("Content-Type", "application/x-www-form-urlencoded")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "GET");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
res = curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
POST
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "[email protected][email protected]&key=<YourAPIkey>";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let config = {
method: 'get',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>',
headers: { }
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
POST
const axios = require('axios');
const qs = require('qs');
let data = qs.stringify({
'email': '[email protected]',
'email': '[email protected]',
'key': '<YourAPIkey>'
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'api.genderapi.io',
'path': '/api/email/?email%5B%[email protected]&email%5B%[email protected]&key=<YourAPIkey>',
'headers': { },
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
POST
var https = require('follow-redirects').https;
var fs = require('fs');
var qs = require('querystring');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = qs.stringify({
'email': '[email protected]',
'email': '[email protected]',
'key': '<YourAPIkey>'
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>',
'headers': { }
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
POST
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/email/',
'headers': {
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'email': '[email protected]',
'email': '[email protected]',
'key': '<YourAPIkey>'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('GET', 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
POST
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/email/')
.headers({
'Content-Type': 'application/x-www-form-urlencoded'
})
.send('[email protected]')
.send('[email protected]')
.send('key=<YourAPIkey>')
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/?email%5B%[email protected]&email%5B%[email protected]&key=<YourAPIkey>"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"GET"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
POST
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/x-www-form-urlencoded"
};
[request setAllHTTPHeaderFields:headers];
NSMutableData *postData = [[NSMutableData alloc] initWithData:[@"[email protected]" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"[email protected]" dataUsingEncoding:NSUTF8StringEncoding]];
[postData appendData:[@"&key=<YourAPIkey>" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>" in
Client.call `GET uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
POST
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "[email protected][email protected]&key=<YourAPIkey>";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/x-www-form-urlencoded"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
POST
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => '[email protected][email protected]&key=<YourAPIkey>',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/x-www-form-urlencoded'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$request = new Request('GET', 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>');
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
POST
$client = new Client();
$headers = [
'Content-Type' => 'application/x-www-form-urlencoded'
];
$options = [
'form_params' => [
'email' => '[email protected]',
'email' => '[email protected]',
'key' => '<YourAPIkey>'
]];
$request = new Request('POST', 'https://api.genderapi.io/api/email/', $headers);
$res = $client->sendAsync($request, $options)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>');
$request->setMethod(HTTP_Request2::METHOD_GET);
$request->setConfig(array(
'follow_redirects' => TRUE
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
POST
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$request->addPostParameter(array(
'email' => '[email protected]',
'email' => '[email protected]',
'key' => '<YourAPIkey>'
));
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>');
$request->setRequestMethod('GET');
$request->setOptions(array());
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
POST
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append(new http\QueryString(array(
'email' => '[email protected]',
'email' => '[email protected]',
'key' => '<YourAPIkey>')));$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/x-www-form-urlencoded'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>' -Method 'GET' -Headers $headers
$response | ConvertTo-Json
POST
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "[email protected][email protected]&key=<YourAPIkey>"
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = ''
headers = {}
conn.request("GET", "/api/email/?email%5B%[email protected]&email%5B%[email protected]&key=<YourAPIkey>", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
POST
import http.client
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = '[email protected][email protected]&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/api/email/", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
url = "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>"
payload = {}
headers = {}
response = requests.request("GET", url, headers=headers, data=payload)
print(response.text)
POST
import requests
url = "https://api.genderapi.io/api/email/"
payload = '[email protected][email protected]&key=<YourAPIkey>'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
library(httr)
res <- VERB("GET", url = "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")
cat(content(res, 'text'))
POST
library(httr)
headers = c(
'Content-Type' = 'application/x-www-form-urlencoded'
)
body = list(
'email' = '[email protected]',
'email' = '[email protected]',
'key' = '<YourAPIkey>'
)
res <- VERB("POST", url = "https://api.genderapi.io/api/email/", body = body, add_headers(headers), encode = 'form')
cat(content(res, 'text'))
library(RCurl)
res <- getURL("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>", .opts=list(followlocation = TRUE))
cat(res)
POST
library(RCurl)
headers = c(
"Content-Type" = "application/x-www-form-urlencoded"
)
params = c(
"email" = "[email protected]",
"email" = "[email protected]",
"key" = "<YourAPIkey>"
)
res <- postForm("https://api.genderapi.io/api/email/", .params = params, .opts=list(httpheader = headers, followlocation = TRUE), style = "post")
cat(res)
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Get.new(url)
response = https.request(request)
puts response.read_body
POST
require "uri"
require "net/http"
url = URI("https://api.genderapi.io/api/email/")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/x-www-form-urlencoded"
request.body = "[email protected][email protected]&key=<YourAPIkey>"
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let request = client.request(reqwest::Method::GET, "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>");
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
POST
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse()?);
let mut params = std::collections::HashMap::new();
params.insert("email", "[email protected]");
params.insert("email", "[email protected]");
params.insert("key", "<YourAPIkey>");
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/email/")
.headers(headers)
.form(¶ms);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
http --follow --timeout 3600 GET 'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>'
POST
http --ignore-stdin --form --follow --timeout 3600 POST 'https://api.genderapi.io/api/email/' \
'email'='[email protected]' \
'email'='[email protected]' \
'key'='<YourAPIkey>' \
Content-Type:'application/x-www-form-urlencoded'
wget --no-check-certificate --quiet \
--method GET \
--timeout=0 \
--header '' \
'https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>'
POST
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/x-www-form-urlencoded' \
--body-data '[email protected][email protected]&key=<YourAPIkey>' \
'https://api.genderapi.io/api/email/'
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/?email[][email protected]&email[][email protected]&key=<YourAPIkey>")!,timeoutInterval: Double.infinity)
request.httpMethod = "GET"
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
POST
let parameters = "[email protected][email protected]&key=<YourAPIkey>"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/")!,timeoutInterval: Double.infinity)
request.addValue("application/x-www-form-urlencoded", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 2,
"remaining_credits": 7276,
"expires": 1717069765,
"q": "[email protected];[email protected]",
"names": [
{
"name": "anna",
"q": "[email protected]",
"gender": "female",
"country": "US",
"total_names": 80455,
"probability": 99
},
{
"name": "james",
"q": "[email protected]",
"gender": "male",
"country": "US",
"total_names": 70373,
"probability": 99
}
],
"duration": "4ms"
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
names | Array | Any | List of your results |
duration | String | milliseconds("4ms") | Processing time of your request on server |
names(Array) | |||
---|---|---|---|
Field | Data Type | Value | Description |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |
Multiple Emails with Country Filter
In one country, a name can be female while in another country, it can be male.
By adding the country filter to your queries, you can obtain gender information specific to that country.
Find the ISO 3166-1 alpha-2 country codes
* If there is no result with given country filter, we will give the global name result.
* You can query multiple emails at once. The maximum limit is 50.
POST /api/email/multi/country HTTP/1.1
Host: api.genderapi.io
Content-Type: application/json
{
"key":"<YourAPIkey>",
"data":[
{"email":"[email protected]","country":"DE"},
{"email":"[email protected]","country":"IT"},
{"email":"[email protected]","country":"US"}
]
}
Select Your Programing Language
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.genderapi.io/api/email/multi/country");
var content = new StringContent("{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}", null, "application/json");
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
Console.WriteLine(await response.Content.ReadAsStringAsync());
var options = new RestClientOptions("https://api.genderapi.io")
{
MaxTimeout = -1,
};
var client = new RestClient(options);
var request = new RestRequest("/api/email/multi/country", Method.Post);
request.AddHeader("Content-Type", "application/json");
var body = @"{" + "\n" +
@" ""key"":""YourApiKey""," + "\n" +
@" ""data"":[{""email"":""[email protected]"",""country"":""DE""},{""email"":""[email protected]"",""country"":""IT""},{""email"":""[email protected]"",""country"":""US""}]" + "\n" +
@"}";
request.AddStringBody(body, DataFormat.Json);
RestResponse response = await client.ExecuteAsync(request);
Console.WriteLine(response.Content);
curl --location 'https://api.genderapi.io/api/email/multi/country' \
--header 'Content-Type: application/json' \
--data '{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}'
var headers = {
'Content-Type': 'application/json'
};
var data = json.encode({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
var dio = Dio();
var response = await dio.request(
'https://api.genderapi.io/api/email/multi/country',
options: Options(
method: 'POST',
headers: headers,
),
data: data,
);
if (response.statusCode == 200) {
print(json.encode(response.data));
}
else {
print(response.statusMessage);
}
var headers = {
'Content-Type': 'application/json'
};
var request = http.Request('POST', Uri.parse('https://api.genderapi.io/api/email/multi/country'));
request.body = json.encode({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
request.headers.addAll(headers);
http.StreamedResponse response = await request.send();
if (response.statusCode == 200) {
print(await response.stream.bytesToString());
}
else {
print(response.reasonPhrase);
}
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.genderapi.io/api/email/multi/country"
method := "POST"
payload := strings.NewReader(`{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}`)
client := &http.Client { }
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/json")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}");
Request request = new Request.Builder()
.url("https://api.genderapi.io/api/email/multi/country")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.genderapi.io/api/email/multi/country")
.header("Content-Type", "application/json")
.body("{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}")
.asString();
const myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
const raw = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
const requestOptions = {
method: "POST",
headers: myHeaders,
body: raw,
redirect: "follow"
};
fetch("https://api.genderapi.io/api/email/multi/country", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var settings = {
"url": "https://api.genderapi.io/api/email/multi/country",
"method": "POST",
"timeout": 0,
"headers": {
"Content-Type": "application/json"
},
"data": JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
// WARNING: For POST requests, body is set to null by browsers.
var data = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
var xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function() {
if(this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://api.genderapi.io/api/email/multi/country");
xhr.setRequestHeader("Content-Type", "application/json");
xhr.send(data);
val client = OkHttpClient()
val mediaType = "application/json".toMediaType()
val body = "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}".toRequestBody(mediaType)
val request = Request.Builder()
.url("https://api.genderapi.io/api/email/multi/country")
.post(body)
.addHeader("Content-Type", "application/json")
.build()
val response = client.newCall(request).execute()
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(curl, CURLOPT_URL, "https://api.genderapi.io/api/email/multi/country");
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_DEFAULT_PROTOCOL, "https");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/json");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
const char *data = "{\n \"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
res = curl_easy_perform(curl);
curl_slist_free_all(headers);
}
curl_easy_cleanup(curl);
const axios = require('axios');
let data = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
let config = {
method: 'post',
maxBodyLength: Infinity,
url: 'https://api.genderapi.io/api/email/multi/country',
headers: {
'Content-Type': 'application/json'
},
data : data
};
axios.request(config)
.then((response) => {
console.log(JSON.stringify(response.data));
})
.catch((error) => {
console.log(error);
});
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'POST',
'hostname': 'api.genderapi.io',
'path': '/api/email/multi/country',
'headers': {
'Content-Type': 'application/json'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
var postData = JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
});
req.write(postData);
req.end();
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.genderapi.io/api/email/multi/country',
'headers': {
'Content-Type': 'application/json'
},
body: JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var unirest = require('unirest');
var req = unirest('POST', 'https://api.genderapi.io/api/email/multi/country')
.headers({
'Content-Type': 'application/json'
})
.send(JSON.stringify({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
}))
.end(function (res) {
if (res.error) throw new Error(res.error);
console.log(res.raw_body);
});
#import <Foundation/Foundation.h>
dispatch_semaphore_t sema = dispatch_semaphore_create(0);
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.genderapi.io/api/email/multi/country"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
NSDictionary *headers = @{
@"Content-Type": @"application/json"
};
[request setAllHTTPHeaderFields:headers];
NSData *postData = [[NSData alloc] initWithData:[@"{\n \"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}" dataUsingEncoding:NSUTF8StringEncoding]];
[request setHTTPBody:postData];
[request setHTTPMethod:@"POST"];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
dispatch_semaphore_signal(sema);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSError *parseError = nil;
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:0 error:&parseError];
NSLog(@"%@",responseDictionary);
dispatch_semaphore_signal(sema);
}
}];
[dataTask resume];
dispatch_semaphore_wait(sema, DISPATCH_TIME_FOREVER);
open Lwt
open Cohttp
open Cohttp_lwt_unix
let postData = ref "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}";;
let reqBody =
let uri = Uri.of_string "https://api.genderapi.io/api/email/multi/country" in
let headers = Header.init ()
|> fun h -> Header.add h "Content-Type" "application/json"
in
let body = Cohttp_lwt.Body.of_string !postData in
Client.call ~headers ~body `POST uri >>= fun (_resp, body) ->
body |> Cohttp_lwt.Body.to_string >|= fun body -> body
let () =
let respBody = Lwt_main.run reqBody in
print_endline (respBody)
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.genderapi.io/api/email/multi/country',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$client = new Client();
$headers = [
'Content-Type' => 'application/json'
];
$body = '{
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
}';
$request = new Request('POST', 'https://api.genderapi.io/api/email/multi/country', $headers, $body);
$res = $client->sendAsync($request)->wait();
echo $res->getBody();
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.genderapi.io/api/email/multi/country');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'Content-Type' => 'application/json'
));
$request->setBody('{\n "key":"<YourAPIkey>",\n "data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
$client = new http\Client;
$request = new http\Client\Request;
$request->setRequestUrl('https://api.genderapi.io/api/email/multi/country');
$request->setRequestMethod('POST');
$body = new http\Message\Body;
$body->append('{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}');
$request->setBody($body);
$request->setOptions(array());
$request->setHeaders(array(
'Content-Type' => 'application/json'
));
$client->enqueue($request)->send();
$response = $client->getResponse();
echo $response->getBody();
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/json")
$body = @"
{
`"key`":`"<YourAPIkey>`",
`"data`":[{`"email`":`"[email protected]`",`"country`":`"DE`"},{`"email`":`"[email protected]`",`"country`":`"IT`"},{`"email`":`"[email protected]`",`"country`":`"US`"}]
}
"@
$response = Invoke-RestMethod 'https://api.genderapi.io/api/email/multi/country' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
import http.client
import json
conn = http.client.HTTPSConnection("api.genderapi.io")
payload = json.dumps({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
})
headers = {
'Content-Type': 'application/json'
}
conn.request("POST", "/api/email/multi/country", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
import requests
import json
url = "https://api.genderapi.io/api/email/multi/country"
payload = json.dumps({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
library(httr)
headers = c(
'Content-Type' = 'application/json'
)
body = '{
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
}';
res <- VERB("POST", url = "https://api.genderapi.io/api/email/multi/country", body = body, add_headers(headers))
cat(content(res, 'text'))
library(RCurl)
headers = c(
"Content-Type" = "application/json"
)
params = "{
\"key\": \"<YourAPIkey>\",
\"data\": [
{
\"email\": \"[email protected]\",
\"country\": \"DE\"
},
{
\"email\": \"[email protected]\",
\"country\": \"IT\"
},
{
\"email\": \"[email protected]\",
\"country\": \"US\"
}
]
}"
res <- postForm("https://api.genderapi.io/api/email/multi/country", .opts=list(postfields = params, httpheader = headers, followlocation = TRUE), style = "httppost")
cat(res)
require "uri"
require "json"
require "net/http"
url = URI("https://api.genderapi.io/api/email/multi/country")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Content-Type"] = "application/json"
request.body = JSON.dump({
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
})
response = https.request(request)
puts response.read_body
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let client = reqwest::Client::builder()
.build()?;
let mut headers = reqwest::header::HeaderMap::new();
headers.insert("Content-Type", "application/json".parse()?);
let data = r#"{
"key": "<YourAPIkey>",
"data": [
{
"email": "[email protected]",
"country": "DE"
},
{
"email": "[email protected]",
"country": "IT"
},
{
"email": "[email protected]",
"country": "US"
}
]
}"#;
let json: serde_json::Value = serde_json::from_str(&data)?;
let request = client.request(reqwest::Method::POST, "https://api.genderapi.io/api/email/multi/country")
.headers(headers)
.json(&json);
let response = request.send().await?;
let body = response.text().await?;
println!("{}", body);
Ok(())
}
printf '{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}'| http --follow --timeout 3600 POST 'https://api.genderapi.io/api/email/multi/country' \
Content-Type:'application/json'
wget --no-check-certificate --quiet \
--method POST \
--timeout=0 \
--header 'Content-Type: application/json' \
--body-data '{
"key":"<YourAPIkey>",
"data":[{"email":"[email protected]","country":"DE"},{"email":"[email protected]","country":"IT"},{"email":"[email protected]","country":"US"}]
}' \
'https://api.genderapi.io/api/email/multi/country'
let parameters = "{\n\t\"key\":\"<YourAPIkey>\",\n \"data\":[{\"email\":\"[email protected]\",\"country\":\"DE\"},{\"email\":\"[email protected]\",\"country\":\"IT\"},{\"email\":\"[email protected]\",\"country\":\"US\"}]\n}"
let postData = parameters.data(using: .utf8)
var request = URLRequest(url: URL(string: "https://api.genderapi.io/api/email/multi/country")!,timeoutInterval: Double.infinity)
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
return
}
print(String(data: data, encoding: .utf8)!)
}
task.resume()
RESPONSE
{
"status": true,
"used_credits": 3,
"remaining_credits": 7282,
"expires": 1717069765,
"duration": "6ms",
"names": [
{
"name": "andrea",
"q": "[email protected]",
"gender": "female",
"country": "DE",
"total_names": 644,
"probability": 88
},
{
"name": "andrea",
"q": "[email protected]",
"gender": "male",
"country": "IT",
"total_names": 13537,
"probability": 98
},
{
"name": "james",
"q": "[email protected]",
"gender": "male",
"country": "US",
"total_names": 45274,
"probability": 100
}
]
}
Field | Data Type | Value | Description |
---|---|---|---|
status | Boolean | true,false | Please check error codes when false |
used_credits | Integer | Any | Number of credits used on recent request |
remaining_credits | Integer | Any | Number of credits remaining on your package |
expires | Integer | Any | Expiration date of your package in timestamp as seconds |
names | Array | Any | List of your results |
duration | String | milliseconds("4ms") | Processing time of your request on server |
names(Array) | |||
---|---|---|---|
Field | Data Type | Value | Description |
q | String | Any | Your query |
name | String | Any | The found name |
gender | enum[String] | ["male","female","null"] | Result of the gender |
country | enum[String] | ["US","DE","FR","IT",...] | The most encountered country |
total_names | Integer | Any | The number of samples |
probability | Integer | 50 - 100 | The percentage likelihood of the result |